Yes, there’s an OOTB functionality
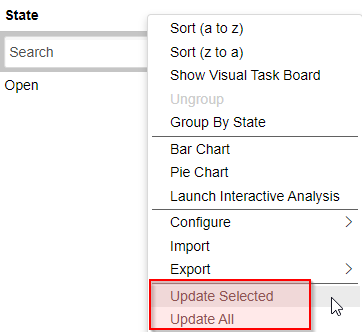
Problem is: it will use the current “view” and thus, most likely, allow to change all fields.
What if you want to restrict this update to certain fields only?
First design a new “view” only containing the fields they are allowed to change on mass. It’s actually a Form Design.
Configure Form Design\New…
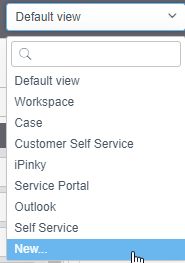
After creation you can switch to the newly created view (or form)
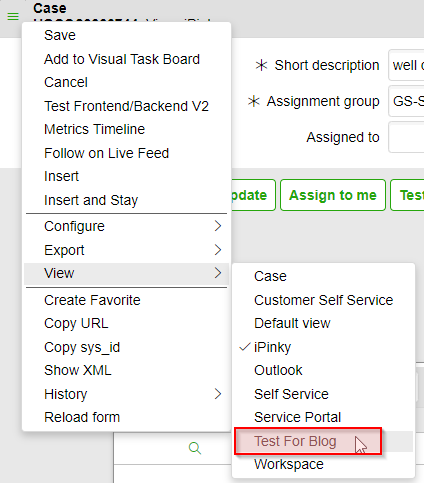
Before: Standard (or default form)
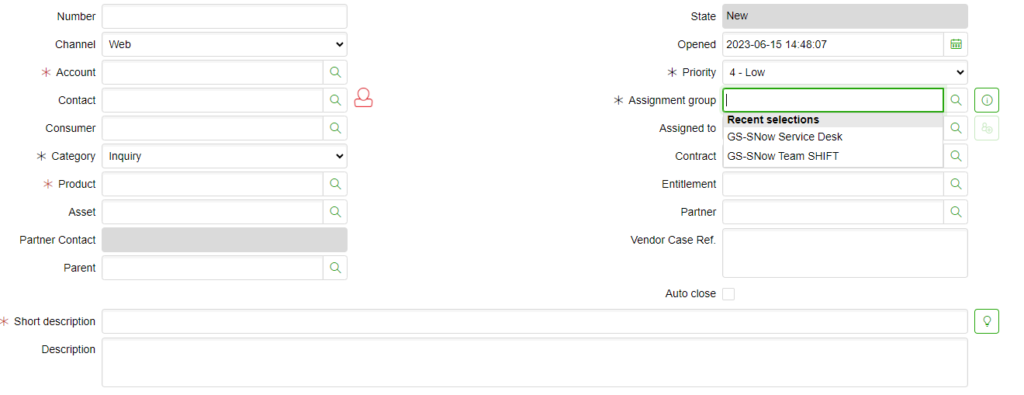
After: my stripped down form

Basic idea:
An Client side UI action where we call the dialog, call the new “submit” button, handle updates on selected records.
Code: doStuff() is set in the Onclick field of the UI action
function doStuff() {
try {
var d = new GlideModalForm('my dialog`s title', 'your_table_name');
//var d = new GlideDialogForm('my dialog`s title', 'your_table_name');
d.addParm('sysparm_view','Test_For_Blog');
d.addParm("sysparm_view_forced", true);
d.addParm('sysparm_form_only','true');
// in the fixSubmit we replace the submit button with our own "submit" button
d.setOnloadCallback(fixSubmit);
// used for GlideDialogForm
//d.setLoadCallback(fixSubmit);
d.render();
} catch (e) {
// log your error
}
}
// this function will be called from the newly created button
// use this function to update your selected records as you please
function mySubmit() {
try {
// document from dialog in iframe
var doc = document.getElementById('dialog_frame').contentWindow.document;
// retrieve values entered in the dialog
var sd = doc.getElementById('sn_customerservice_case.short_description').value;
// g_list.getChecked has a comma separated list of sys_id of records you have selected
var recordsToUpdate = g_list.getChecked().split(',');
recordsToUpdate.forEach(function(thisElement) {
var gr = new GlideRecord('sn_customerservice_case');
// retrieve record
gr.get(thisElement);
// update it's values, maybe add a check if there WAS a value in the dialog, otherwise you'll write '' back to the records
gr.setValue('short_description', sd);
gr.update();
});
// remove the dialog from the DOM, to prevent a nasty message telling you you might lose entered data
document.getElementById('dialog_frame').remove();
// refresh the list
g_list.refresh();
return true;
} catch (e) {
// log your error
}
}
// removing the original submit button as it creates a record which we don't need
// add our own submit button which calls the mySubmit function which updates records as needed
function fixSubmit() {
try {
// document from dialog in iframe
var doc = document.getElementById('dialog_frame').contentWindow.document
// hide original submit button as it always creates a new record which we don't need
var btnSubmit = doc.getElementById('sysverb_insert_bottom');
btnSubmit.style.display = 'none';
// create our own submit button
var btn = doc.createElement("button");
btn.innerHTML = "our own Submit button";
btn.type = "button";
btn.name = "formBtn";
btn.id = "mySubmitButton";
// the mySubmit will not be on the iframe of the dialog but on the entire page
// that's why we need to call it via parent.
btn.setAttribute('onClick', 'parent.mySubmit()');
// insert the newly created button where the original has been hidden above
btnSubmit.parentNode.insertBefore(btn, btnSubmit.nextSibling);
} catch (e) {
// log your error
}
}
The crucial thing to make d.addParm(‘sysparm_view’,’Test_For_Blog’); work was the next line:
d.addParm(“sysparm_view_forced”, true);